Bitcoin Consensus Rules Explained Validating Transactions and Blocks
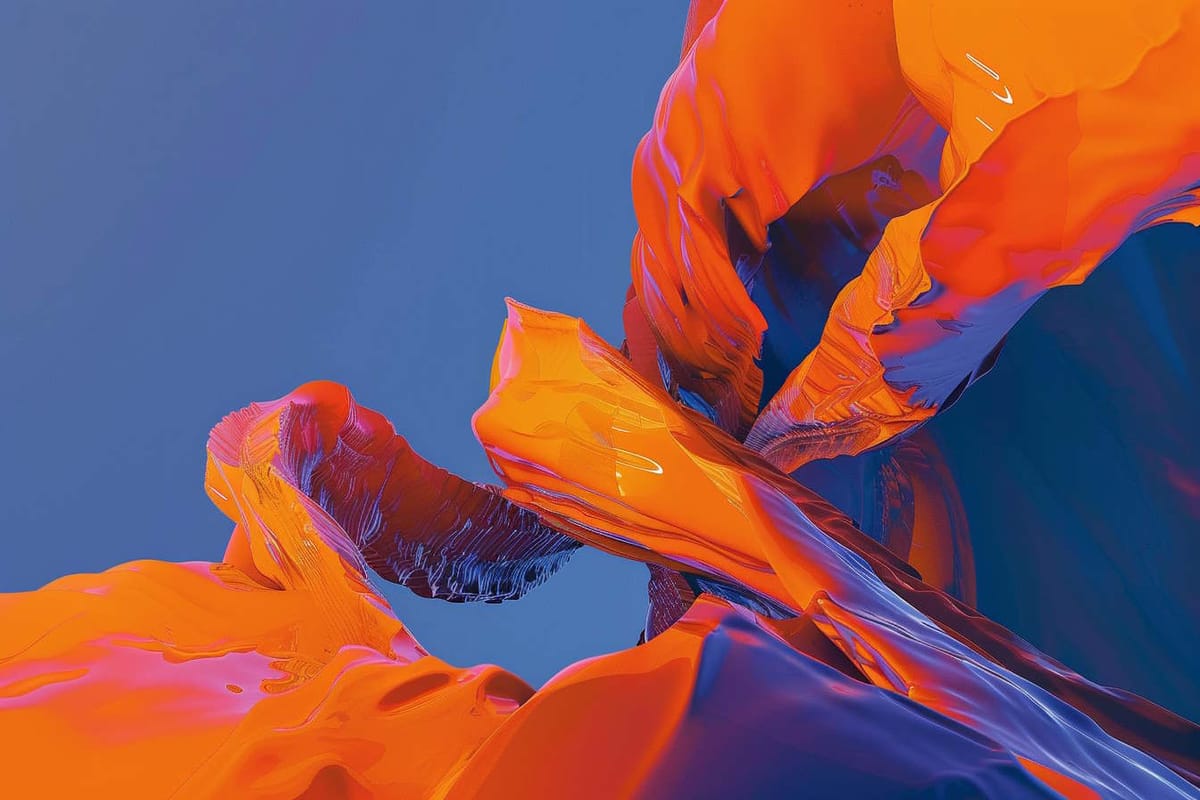
Bitcoin's decentralized nature relies on a set of consensus rules that all nodes in the network must follow. These rules ensure that every node has the same view of the blockchain and that only valid transactions and blocks are added to the ledger.
Consensus rules are essential for maintaining the integrity and security of the Bitcoin network. They prevent double-spending, ensure the proper issuance of new bitcoins, and help nodes reach an agreement on the state of the blockchain.
When a node receives a new transaction, it must validate the transaction before adding it to its mempool and propagating it to other nodes. The validation process involves checking various aspects of the transaction, such as:
- Syntax: The transaction must be properly formatted and contain all required fields
- Signatures: The transaction must be signed with a valid signature that proves ownership of the input funds
- Input UTXOs: The transaction's inputs must reference existing, unspent transaction outputs (UTXOs)
- Output values: The sum of the transaction's output values must not exceed the sum of its input values
Here's a simplified example of a transaction validation function in Python:
# Check transaction syntax
if not is_valid_syntax(transaction):
return False
# Check transaction signatures
if not are_valid_signatures(transaction):
return False
# Check input UTXOs
for input in transaction.inputs:
if not is_unspent(input.utxo):
return False
# Check output values
total_input = sum(input.value for input in transaction.inputs)
total_output = sum(output.value for output in transaction.outputs)
if total_output > total_input:
return False
return True```
In this example, the validate_transaction
function performs various checks on a transaction and returns True
if the transaction is valid or False
otherwise.
Similarly, when a node receives a new block, it must validate the block before adding it to its local copy of the blockchain. The block validation process involves checking:
- Block syntax: The block must be properly formatted and contain all required fields
- Proof-of-Work: The block's hash must meet the current difficulty target
- Timestamp: The block's timestamp must be greater than the median timestamp of the previous 11 blocks
- Block size: The block's size must not exceed the maximum allowed size (currently 1 MB)
- Transactions: All transactions in the block must be valid and not already spent
Here's a simplified example of a block validation function in Python:
# Check block syntax
if not is_valid_syntax(block):
return False
# Check Proof-of-Work
if not is_valid_proof_of_work(block):
return False
# Check timestamp
median_time = median_timestamp(previous_block, 11)
if block.timestamp <= median_time:
return False
# Check block size
if block.size > MAX_BLOCK_SIZE:
return False
# Check transactions
for transaction in block.transactions:
if not validate_transaction(transaction):
return False
return True```
In this example, the validate_block
function performs various checks on a block and its transactions, ensuring that the block adheres to the consensus rules.
If a node receives a transaction or block that violates the consensus rules, it will reject the data and not propagate it to other nodes. This helps maintain the integrity of the Bitcoin network and prevents the spread of invalid data.
By following the same consensus rules, all nodes in the network can independently validate transactions and blocks, ensuring that the blockchain remains consistent and tamper-proof.
As the Bitcoin protocol evolves, the consensus rules may be updated through soft forks or hard forks to introduce new features or address security issues. However, the core principles of transaction and block validation remain crucial for the proper functioning of the network.